Playwright基礎篇(五):基本操作方法
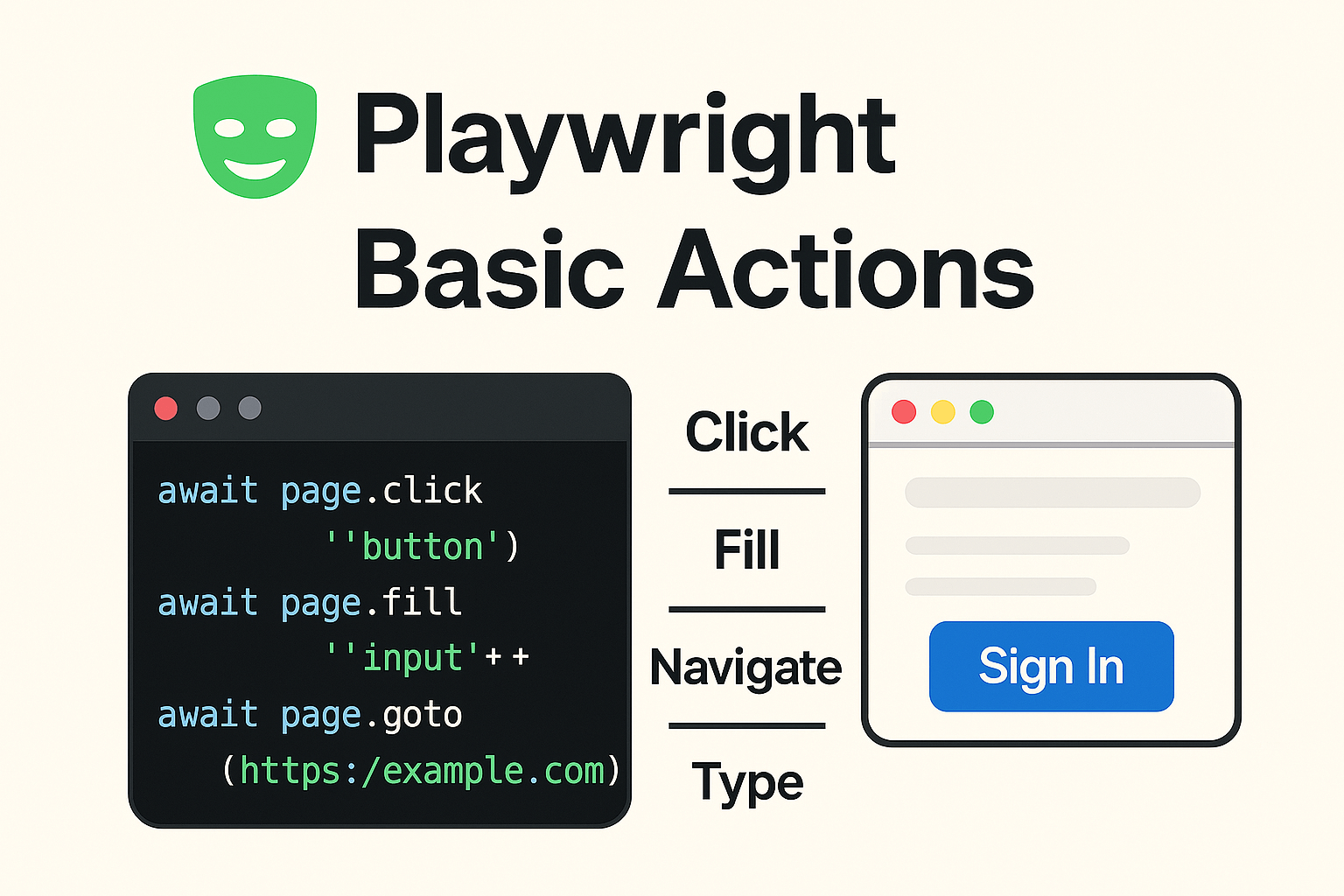
點擊操作 (click)
點擊是與網頁互動最基本的操作。Playwright 提供了多種點擊方式以應對不同場景:
基本點擊:
await page.click('#submit-button');
強制點擊(當元素被其他元素覆蓋時):
await page.click('#hidden-button', { force: true });
多次點擊:
await page.click('#increment', { clickCount: 3 });
精確位置點擊(對於 Canvas 等特殊元素):
await page.click('#drawing-area', { position: { x: 100, y: 150 } });
點擊並等待導航:
await Promise.all([
page.waitForNavigation(),
page.click('#nav-link')
]);
填寫表單 (fill)
fill
方法可快速清除並填入文字內容:
基本填寫:
await page.fill('#username', 'testuser');
填寫並等待變化:
await page.fill('#search-input', 'playwright');
await page.waitForSelector('.search-results');
密碼輸入:
await page.fill('input[type="password"]', 'securePassword123');
清空輸入框:
await page.fill('#comments', '');
選擇下拉選單 (select)
處理下拉選單是常見的互動需求:
根據值選擇:
await page.selectOption('select#country', 'tw');
根據標籤選擇:
await page.selectOption('select#country', { label: '台灣' });
多選(適用於 multiple
屬性的選擇器):
await page.selectOption('select#interests', ['music', 'sports', 'reading']);
選擇第 n 個選項:
await page.selectOption('select#year', { index: 2 });
驗證選擇結果:
const selectedValue = await page.$eval('select#country', select => select.value);
拖曳操作 (drag)
拖曳操作可用於處理排序、拖放上傳等場景:
基本拖曳:
await page.dragAndDrop('#source', '#target');
精確拖曳路徑:
await page.mouse.move(100, 100);
await page.mouse.down();
await page.mouse.move(200, 200);
await page.mouse.up();
拖曳排序:
await page.dragAndDrop('#item1', '#item3');
鍵盤輸入 (press, type)
`keyboard` API 提供了豐富的鍵盤模擬功能:
按下單個鍵:
await page.press('#search-input', 'Enter');
輸入文字(不會清除原有內容):
await page.type('#editor', 'Hello World');
組合鍵操作:
await page.keyboard.press('Control+a');
await page.keyboard.press('Control+c');
按鍵序列:
await page.keyboard.type('Hello');
await page.keyboard.press('Tab');
await page.keyboard.type('World');
進階互動技巧
模擬滑鼠懸停
基本懸停:
await page.hover('.menu-item');
懸停然後點擊子菜單:
await page.hover('.dropdown-trigger');
await page.click('.dropdown-menu .submenu-item');
懸停顯示工具提示並驗證:
await page.hover('.info-icon');
await expect(page.locator('.tooltip')).toBeVisible();
模擬右鍵操作
基本右鍵操作:
await page.click('.context-area', { button: 'right' });
右鍵後選擇選項:
await page.click('.file-item', { button: 'right' });
await page.click('.context-menu-item:has-text("Delete")');
處理彈出視窗
處理 alert/confirm/prompt
對話框:
page.on('dialog', dialog => dialog.accept('Yes'));
await page.click('#alert-button');
處理新開視窗:
const [newPage] = await Promise.all([
context.waitForEvent('page'),
page.click('#open-window')
]);
await newPage.waitForLoadState();
await newPage.close();
處理彈出框架:
const popupFrame = page.frameLocator('.popup-frame');
await popupFrame.locator('button').click();
檔案下載處理:
const [download] = await Promise.all([
page.waitForEvent('download'),
page.click('#download-button')
]);
const path = await download.path();
總結
Playwright 提供了豐富的互動操作 API,可以模擬幾乎所有的用戶行為。掌握這些基本和進階互動技巧,可以幫助我們構建健壯的自動化測試和網頁爬蟲。無論是簡單的點擊填寫,還是複雜的拖曳、右鍵操作,Playwright 都能夠精確模擬真實用戶的操作行為。
分享這篇文章: