Playwright進階篇(六):使用 Allure Report 整合測試報告
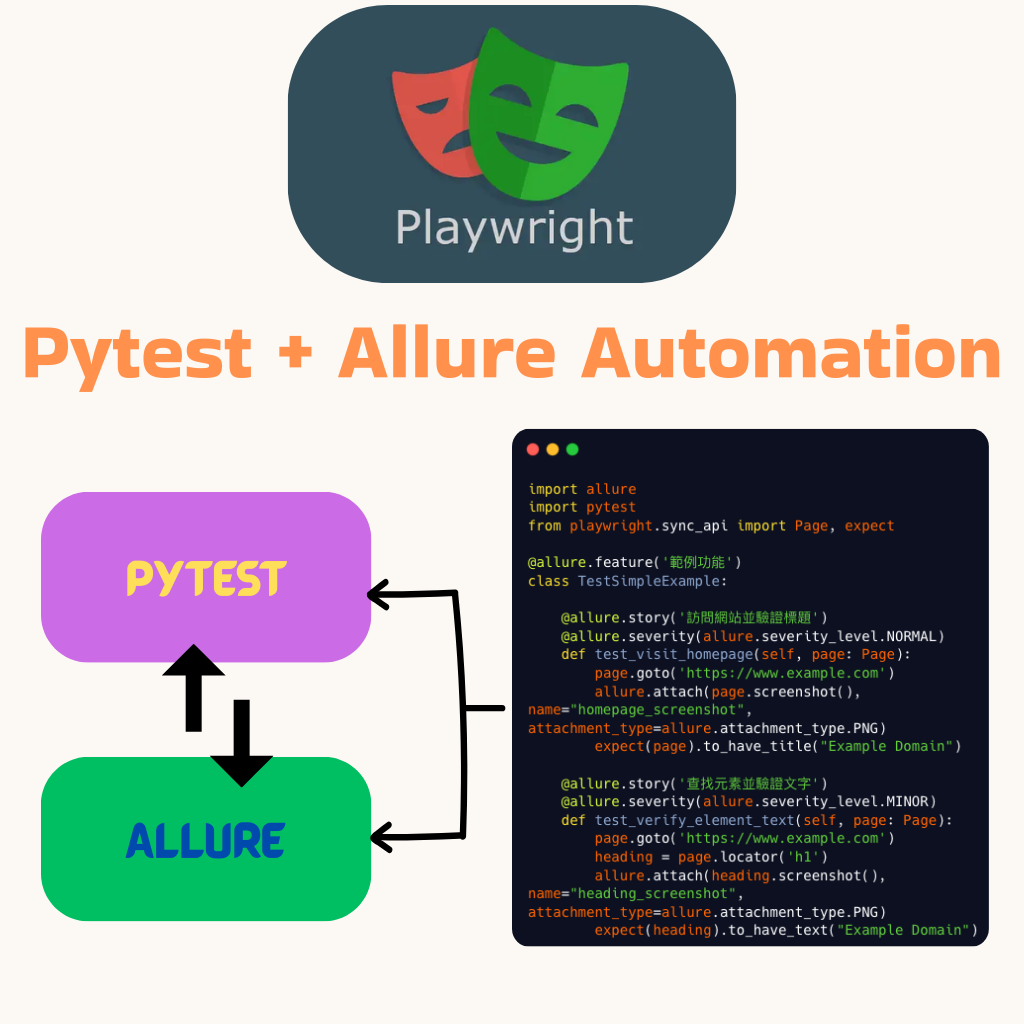
1. 環境設置
1.1 建立虛擬環境
python3 -m venv venv
source venv/bin/activate
1.2 安裝必要套件
pip install playwright pytest-playwright allure-pytest
1.3 安裝 Playwright 瀏覽器
playwright install
1.4 安裝 Allure 命令行工具(macOS)
brew install allure
2. 基本測試結構
2.1 建立測試文件
建立 test_example.py
文件,基本結構如下:
import allure
import pytest
from playwright.sync_api import Page, expect
@allure.feature('功能名稱')
class TestExample:
@allure.story('測試場景')
@allure.severity(allure.severity_level.NORMAL)
def test_example(self, page: Page):
# 測試步驟
pass
2.2 常用的 Allure 裝飾器
@allure.feature
: 標記功能模組@allure.story
: 標記測試場景@allure.severity
: 標記測試重要程度- BLOCKER: 阻塞性缺陷
- CRITICAL: 嚴重缺陷
- NORMAL: 普通缺陷
- MINOR: 次要缺陷
- TRIVIAL: 載微缺陷
3. 頁面操作和驗證
3.1 基本頁面操作
# 訪問網頁
page.goto('https://example.com')
# 定位元素
element = page.locator('selector')
# 輸入文字
element.fill('text')
# 點擊元素
element.click()
3.2 使用 expect 進行斷言
# 驗證頁面標題
expect(page).to_have_title("Expected Title")
# 驗證元素文字
expect(page.locator('h1')).to_have_text("Expected Text")
4. 截圖功能
4.1 基本截圖
# 一般截圖
allure.attach(
page.screenshot(),
name="screenshot_name",
attachment_type=allure.attachment_type.PNG
)
4.2 進階截圖選項
# 完整頁面截圖(包含需要滾動的部分)
page.screenshot(full_page=True)
# 元素截圖
element = page.locator('h1')
element.screenshot()
# 自定義區域截圖
page.screenshot(clip={"x": 0, "y": 0, "width": 500, "height": 300})
# 遮蔽元素的截圖
page.screenshot(mask=[page.locator('h1')])
4.3 失敗時自動截圖
try:
# 測試步驟
...
except Exception as e:
allure.attach(
page.screenshot(),
name="failure_screenshot",
attachment_type=allure.attachment_type.PNG
)
raise e
5. 執行測試
5.1 運行測試並生成報告
# 運行測試並生成報告數據
pytest --alluredir=./allure-results
# 查看報告
allure serve allure-results
5.2 報告內容
Allure 報告包含:
- 測試執行狀態概覽
- 詳細的測試步驟
- 測試截圖和其他附件
- 測試執行時間統計
- 按嚴重程度分類的測試案例
- 失敗測試的詳細信息
6. 最佳實踐
- 為每個測試案例添加清晰的描述(使用 Allure 裝飾器)
- 在關鍵步驟添加截圖
- 使用 try-except 捕獲異常並添加失敗截圖
- 合理組織測試案例,使用 feature 和 story 進行分類
- 根據測試的重要性設置適當的嚴重程度
結論
為 Playwright 大型專案設計良好的架構至關重要。透過模組化拆分測試、建立共用元件以提升效率,並實施環境分離確保不同環境的穩定性,我們可以打造出更易於維護、擴展且高效的測試體系。本文所探討的三個核心要素,是構建穩健 Playwright 大型專案測試架構的關鍵,能有效提升測試品質並降低長期維護成本。
分享這篇文章: